Singleton vs Scoped vs Transient
This article describes the service scope in ASP.NET Core and the difference between AddSingleton, AddScoped and AddTransient methods. Let us see the following example that shows the lifetime of the services
The following is an interface. This interface only returns a string unique ID (GuidID).
IRepository.cs
The below code is an implementation of the above interface. It has a constructor MyRepository which creates a unique ID when the object is created. The final one is GetUniqueID() which returns the unique ID.
MyRepository.cs
AddSingleton
AddSingleton will generate an instance when requested for the first time. Then it will use the same instance for further request.
To use the service first, you must register with the ConfigureService method in Startup.cs. This ConfigureServices method enables you to add services to the application. Here I have added AddSingleton service with Service Type IRepository and implementation MyRepository
Startup.cs
The following is a HomeController code. Here the IRepository service is injected in HomeController construction. In the Index action method uniqueID is received from GetUniqueID() method and assigned to ViewData[“UniqueID”]
HomeController.cs
The following is an Index view code. The unique ID in ViewData [“UniqueID”] is shown here and a partial view named UniquePartialView has been added to the view.
Index.cshtml
Next is a partial view code. The service is injected using @Inject directive and the unique ID is shown.
UniquePartialView.cshtml
The following is an output of the singleton. The unique ID here is the same for the parent view and the child view. Also, if you access the same page in the new browser, it will show the same unique ID. Even if you refresh the page it does not change the unique ID.
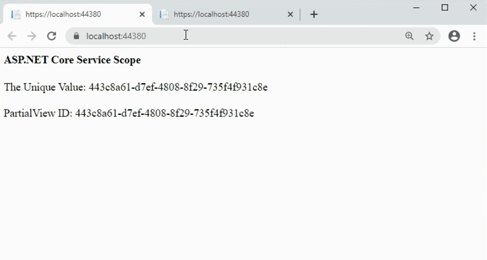
AddScoped
For Scoped, an instance will be created per client request. The following is a ConfigureServices in the Startup.cs. Here I have registered the AddScoped for the above example Paragraph
Startup.cs
The following is an output of AddScoped. The unique ID here is the same for the parent view and partial view. But the unique ID will change when you refresh the page. This means it will create a new instance for each request.
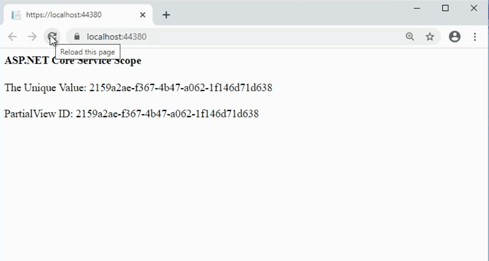
AddTransient
For Transient, the instance will be created for each request. To test AddTransient’s lifetime, I have registered the AddTransient in ConfigureServices method in Startup.cs.
The following is an output of AddTransient. The unique ID here is different for the parent view and partial view. When refreshing the page, it brings up different unique IDs for parent and child view.
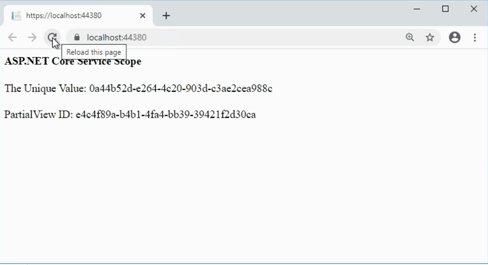
This blog explains what is the lifetime for the service and the difference between AddSingleton, AddScoped and AddTransient.
If you have any questions, please leave a comment below.